What is Redis?
Redis which stands for Remote Dictionary Server is an open-source (BSD licensed), in-memory data structure store, used as a database, cache, and message broker.
In basic words, Redis is a Key-Value Store that stores data in Key-Value pairs.
For Example:
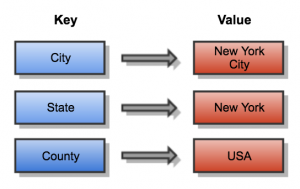
Redis is a No-SQL database.
Basically, this means it does not have tables, rows, columns, functions, procedures, etc like in MySQL, Oracle DBs
It also does not use SELECT, INSERT, UPDATE, DELETE.
Instead, it uses data structures to store data.
Like,
- String
- Lists
- Sorted Sets
- Hashes
- Sets
And,
- Bitmaps (also called bit arrays, bit vectors, etc.)
- Hyperloglogs ( It’s is a probabilistic data structure used to count unique values — or as it’s referred to in mathematics: calculating the cardinality of a set)
- Geospatial Indexes (The geospatial index supports containment and intersection queries for various geometric 2D shapes.)
Interaction with data in Redis is command based.
One of the key differences between Redis and other key-value databases is Redis’s ability to store and manipulate high-level data types.
Redis is a popular choice for caching, session management, gaming, leader boards, real-time analytics, geospatial, ride-hailing, chat/messaging, media streaming, and pub/sub-apps.
To learn more about Redis you can visit their website, redis.io.
Getting Started
For this post, I’ll be working with Ubuntu 18.04 but any Linux Distro works.
You’ll need PHP (Laravel) installed, Follow the instructions here to get it installed https://panjeh.medium.com/install-laravel-on-ubuntu-18-04-with-apache-mysql-php7-lamp-stack-5512bb93ab3f;
For test purposes, You’ll also need Redis installed locally.
To do this simply run,
sudo apt-get update
Then,
sudo apt-get install redis-server
Finally;
sudo systemctl enable redis-server.service
One more thing,
sudo nano /etc/redis/redis.conf
Optionally, you can increase or decrease the memory limit is available on your host machine.
maxmemory 256mb
maxmemory-policy allkeys-lru
Then finally restart your Redis server.
sudo systemctl restart redis-server.service
and start it up by running
redis-server
By default, it runs on host: 127.0.0.1 and port: 6379.
Finally, Getting Started
We’ll be using a test project for our example.
- So open up your terminal with CTRL+ALT+T, and type in the command below in the directory of your choice;
composer create-project — prefer-dist laravel/laravel redis-example
- Navigate to the folder
cd redis-example
Install the predis/predis package via Composer
composer require predis/predis
Configuration
The Redis configuration for your application can be found in config/database.php. In this file, you’ll find a Redis array containing the Redis servers utilized by your application.
'redis' => [
'client' => env('REDIS_CLIENT', 'predis'),
'default' => [
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => env('REDIS_DB', 0),
],
'cache' => [
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => env('REDIS_CACHE_DB', 1),
],
],
The default configuration uses phpredis as it’s client, so you’ll have to change this to predis if you’ll be using the laravel predis package.
Interacting with Redis
You can interact with Redis by calling various methods on the Redis facade.
Redis facade supports dynamic methods, basically, this means that you could call any command on the facade and the command will be passed directly to Redis.
Here’s an example,
namespace App\Http\Controllers; use App\Http\Controllers\Controller; use Illuminate\Support\Facades\Redis; class UserController extends Controller { /** * Show the profile for the given user. * * @param int $id * @return Response */ public function showProfile($id) { Redis::set('name', 'Taylor'); $values = Redis::lrange('names', 5, 10); $name = Redis::get(‘name’.$id); // $values = Redis::command('lrange', ['name', 5, 10]); $user = [ 'name' => $name, 'values' => $values ]; return view(‘user.profile’, [‘user’ => $user]); } }
For more commands like this, you can check out the laravel documentation on Redis here
Source: https://olat-nji.medium.com/getting-started-with-redis-as-a-php-laravel-guy-or-girl-6a8d875a4166