Finally a library you can pick up in under 5 minutes
Costas AndreouDec 6 · 4 min read
The biggest advantage of python is the ease of use and the abundance of libraries for just about anything. With a few lines of code, there is nothing you couldn’t do. As long as your python scripts are for personal use or your target audience is technical enough, you would never even have to think about a User Interface (UI).
Sometimes, however, your target audience is not technical enough. They’d love to use your python scripts but only as long as they didn’t have to look at a single line of code. In those cases, providing command line scripts will simply not cut it. You would ideally need to provide them with a UI. Although I wouldn’t be surprised if you have your typical desktop client versus web-based UI debate, in this blog posts, the aim is to use Python exclusively.
Python Libraries Available for UI usage
There are essentially 3 big Python UI libraries; Tkinter, wxPython and PyQT. While reviewing all three, I realised that everything that I liked about Python was nowhere to be found in using these libraries. Python libraries, in general, make a very good job of abstracting away the super technical. If I needed to work with Object Oriented Programming, I might as well have loaded up Java or .Net.
Much to my delight, however, I came across a fourth option that seemed to be catering to my kind of liking. The library I begun reviewing and ultimately choosing to create Python UIs from is called PySimpleGUI. Funnily enough, this library is using all the 3 popular libraries, but abstracts away the super technical.
Without any further ado, let’s dive in and explore this library by solving a real problem at the same time.
Check that two files are identical
Using my previous article 3 Quick Ways to Compare Data in Python, we can use the first section, Check the Integrity of the Data to attempt to build a UI.3 Quick Ways To Compare Data in PythonFor anyone working in an analytical role, receiving requests to compare data will be all too familiar. Whether that is…medium.com
We essentially need a way to load up two files, and then choose the encryption we would like to use to do the file comparison.
Code the UI
To build that UI, we can use the following code:
import PySimpleGUI as sg
layout = [
[sg.Text('File 1'), sg.InputText(), sg.FileBrowse(),
sg.Checkbox('MD5'), sg.Checkbox('SHA1')
],
[sg.Text('File 2'), sg.InputText(), sg.FileBrowse(),
sg.Checkbox('SHA256')
],
[sg.Output(size=(88, 20))],
[sg.Submit(), sg.Cancel()]
]
window = sg.Window('File Compare', layout)
while True: # The Event Loop
event, values = window.read()
# print(event, values) #debug
if event in (None, 'Exit', 'Cancel'):
break
which results in:
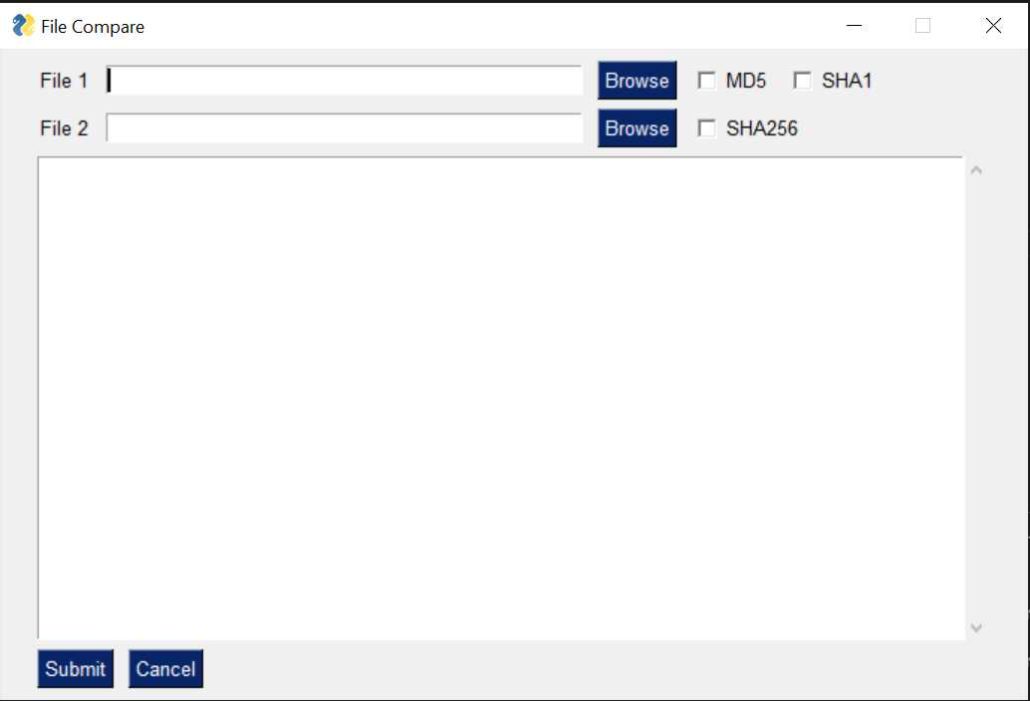
Plugging in the logic
With the UI in place, it’s simple for one to see how to plug in the rest of the code. We simply need to monitor for what the user inputs and then act accordingly. We can very easily do that, with the following code.
import PySimpleGUI as sg
import re
import hashlibdef hash(fname, algo):
if algo == 'MD5':
hash = hashlib.md5()
elif algo == 'SHA1':
hash = hashlib.sha1()
elif algo == 'SHA256':
hash = hashlib.sha256()
with open(fname) as handle: #opening the file one line at a time for memory considerations
for line in handle:
hash.update(line.encode(encoding = 'utf-8'))
return(hash.hexdigest())layout = [
[sg.Text('File 1'), sg.InputText(), sg.FileBrowse(),
sg.Checkbox('MD5'), sg.Checkbox('SHA1')
],
[sg.Text('File 2'), sg.InputText(), sg.FileBrowse(),
sg.Checkbox('SHA256')
],
[sg.Output(size=(88, 20))],
[sg.Submit(), sg.Cancel()]
]window = sg.Window('File Compare', layout)while True: # The Event Loop
event, values = window.read()
# print(event, values) #debug
if event in (None, 'Exit', 'Cancel'):
break
if event == 'Submit':
file1 = file2 = isitago = None
# print(values[0],values[3])
if values[0] and values[3]:
file1 = re.findall('.+:\/.+\.+.', values[0])
file2 = re.findall('.+:\/.+\.+.', values[3])
isitago = 1
if not file1 and file1 is not None:
print('Error: File 1 path not valid.')
isitago = 0
elif not file2 and file2 is not None:
print('Error: File 2 path not valid.')
isitago = 0
elif values[1] is not True and values[2] is not True and values[4] is not True:
print('Error: Choose at least one type of Encryption Algorithm')
elif isitago == 1:
print('Info: Filepaths correctly defined.')
algos = [] #algos to compare
if values[1] == True: algos.append('MD5')
if values[2] == True: algos.append('SHA1')
if values[4] == True: algos.append('SHA256')
filepaths = [] #files
filepaths.append(values[0])
filepaths.append(values[3])
print('Info: File Comparison using:', algos)
for algo in algos:
print(algo, ':')
print(filepaths[0], ':', hash(filepaths[0], algo))
print(filepaths[1], ':', hash(filepaths[1], algo))
if hash(filepaths[0],algo) == hash(filepaths[1],algo):
print('Files match for ', algo)
else:
print('Files do NOT match for ', algo)
else:
print('Please choose 2 files.')window.close()
Running the above code will give you the following outcome:
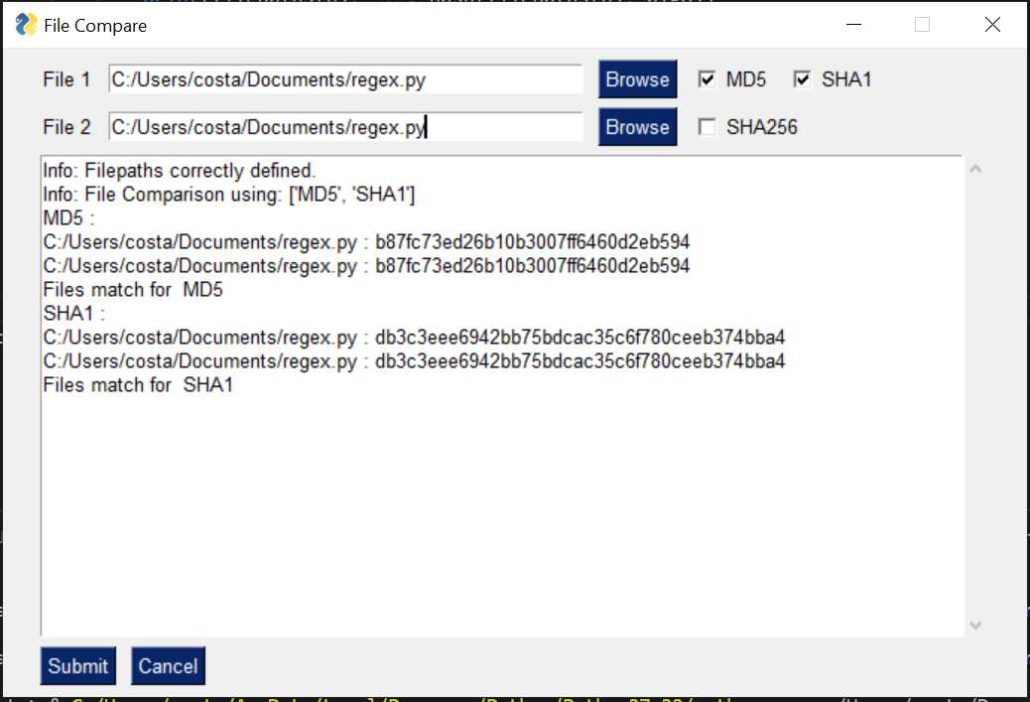
Closing Thoughts
Although not the prettiest of UIs, this library allows you to quickly spin up simple python UIs and share them with whoever you may need to. More importantly, the code that you require to do so, is simple and very readable. You will still have the problem of having to run the code to get the UI, which may make sharing a bit difficult, but you can consider using something like PyInstaller which will turn your python script into a .exe that people can simply double click.
Costas Andreou
Let’s simplify things!
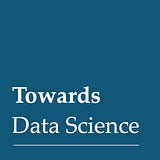
Towards Data Science
More from Towards Data Science
Is Julia Set to Take Over Python the Same Way Python Took Over JAVA?